Chrome debugging: The power of “Store as global variable”
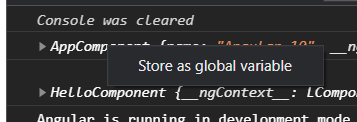
After working on the web for nearly ten years, I forget how many easy tricks I have learned along the way that others could make use of and may not even know about. I will try to post these as I think of them so that maybe you can benefit from them.
This trick has been very helpful to me when dealing with complicated scopes and vendor components within the scope. Chrome has a nifty ability to take whatever you console.log and store it as a global variable.
The power of this lies in the fact that when you store objects this way, it is stored as a reference. This means that anything you modify in the globally stored variable is reflected in the variables specific scope.
To demonstrate this I’ve set up a quick angular project in StackBlitz. We’re just going to do this in the AppComponent of this project. It looks something like this.
import { Component, VERSION, OnInit } from "@angular/core"; @Component({ selector: "my-app", templateUrl: "./app.component.html", styleUrls: ["./app.component.css"] }) export class AppComponent implements OnInit { name = "Angular " + VERSION.major; ngOnInit() { console.log(this); } click() { console.log(this.name); } }
As you can see we are going to log the component class itself when the component initializes. Our application is here https://debugging-test.stackblitz.io/
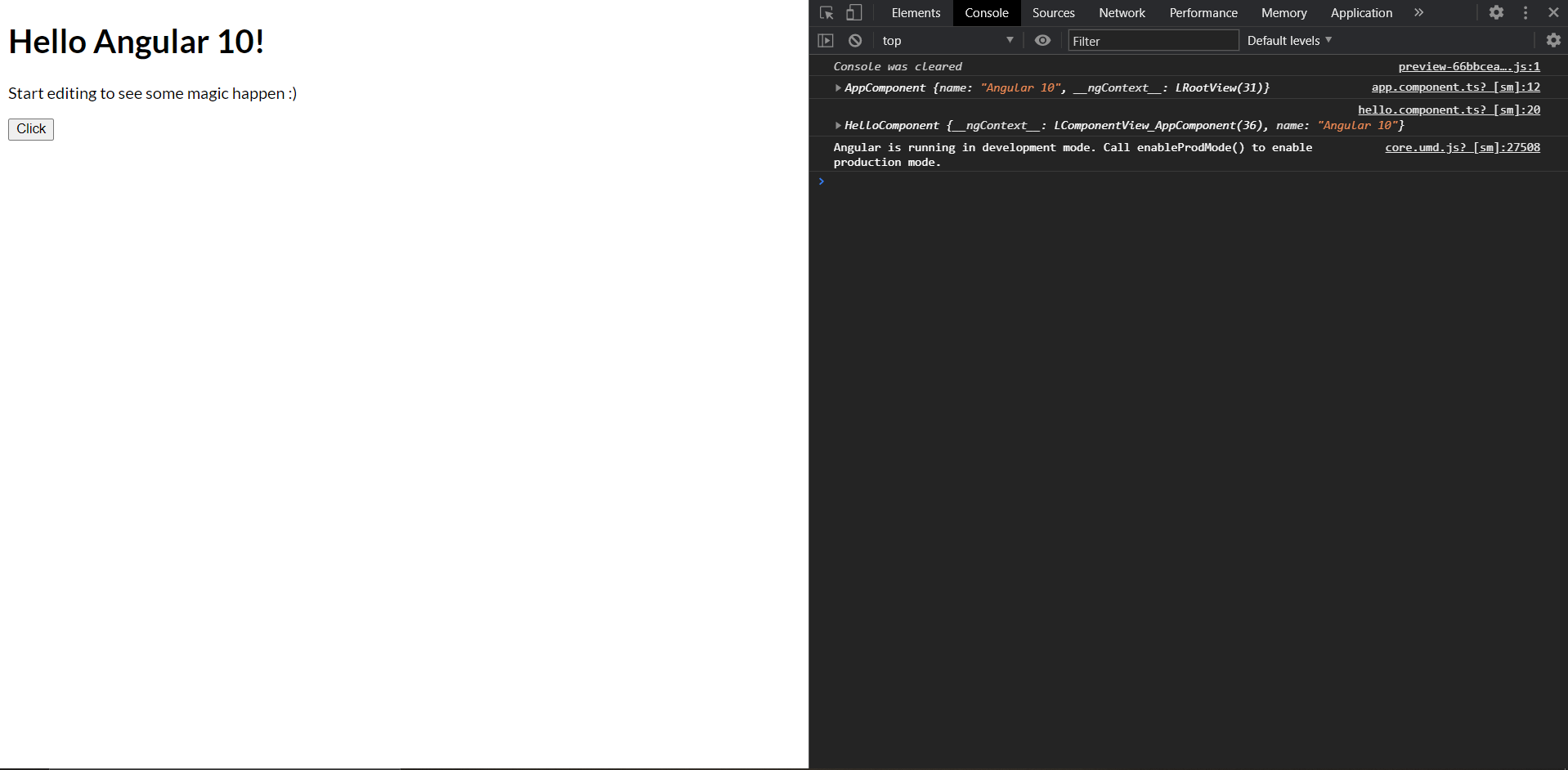
AppComponent is logged in the console. If we right click the output, chrome will give us an option called “Store as global variable”
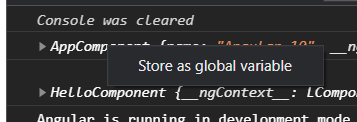
When we click this, it will save it in a variable called “temp1”.
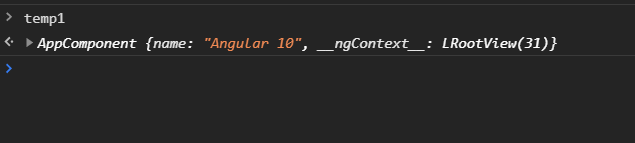
So now that we have that, we’re going to modify the property called “name”.
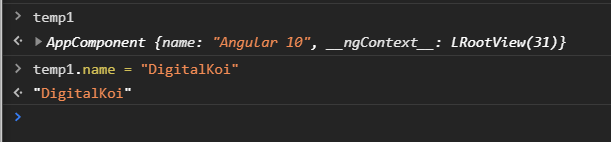
This modifies the property in the global variable and, since it is stored as a reference, it also modifies it in the scope of the AppComponent.
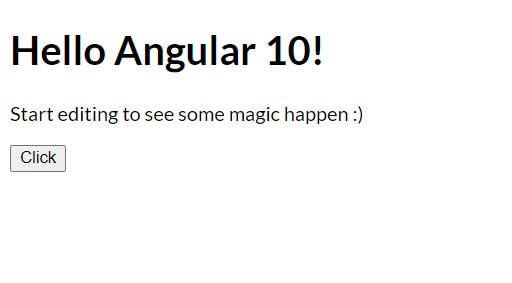
Our name hasn’t updated yet in the component, because the view has not been checked again. This is why we added our click function to log the name out again and trigger the view check.
After we click the button our output looks as follows:
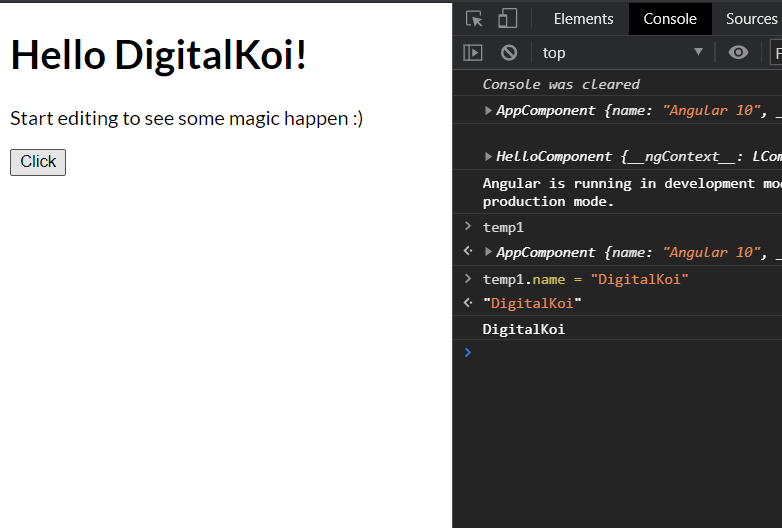